The Project
Our team was tasked with enhancing this addressbook for our Software Engineering Project. We made the decision to morph it into a personalized file manager, PDF++. Inspired by applications that bring an upgrade to the atypical solution like Notepad++, we wanting to make an application relevant for students. This application allows you to set datelines and tags for files, with managing PDFs as its main specialty. It is mainly a CLI (Command Line Interface) application i.e. commands are executed through typing the desired command in the input line provided. However, several commands also allow for input through a GUI (Graphical User Interface), where the user is able to click on the desired input.
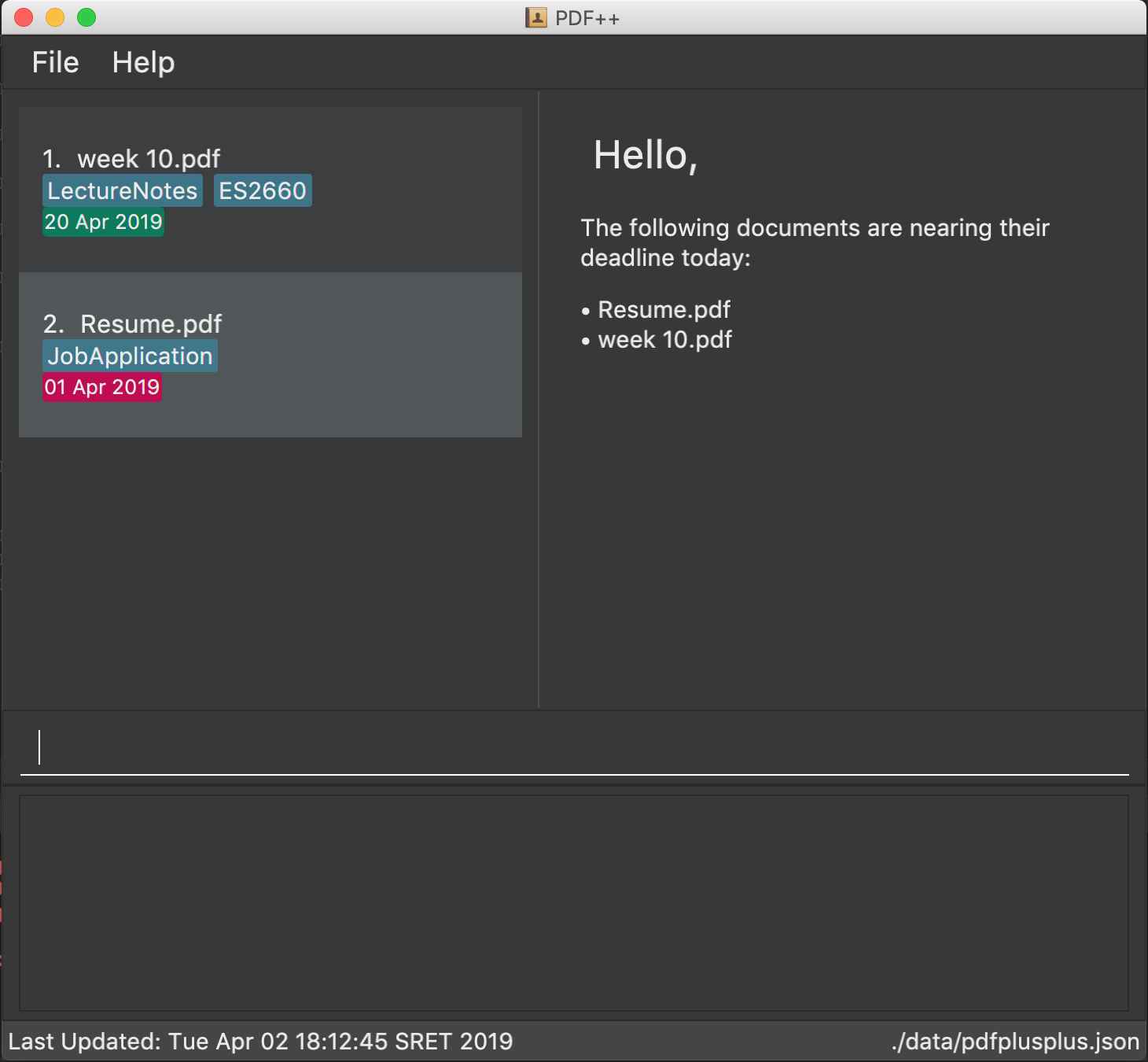
Note the following symbols and formatting used in this document:
Grey highlights
(called mark-ups) indicate that this is a command that
can be executed by the application.
Summary of Contributions
The following section details a summarised overview of my contributions to the team project in the areas of coding, documentation and design.
Enhancements added:
-
merge
command-
Description: I added the ability to append two of more PDF files within the application to so that a new file is created with the merged content.
-
Justification: Merging PDF files is highly utilised, especially by students or users handling numerous documentations. However, merging of PDF files is usually a service provided by paid versions of PDF managers or other external online services.
-
Highlight: Merging can be done quickly through the application, without the inconvenience of relying on other software and online services to do it.
-
Credits: Apache PDFBox® library ( PDFMergerUtility, PDDocument )
-
-
find
command optimisation-
Description: Based on the originial ability of addressbook to find files based on name fully matching search keyword, I added the ability to find files based on partial matching of file name, as well as matching of any text content within the file.
-
Justification: PDF++ is intended to be complete replacement for managing files. For finding a file on most other filesystems, the full name of the file is not required, hence it should also be made the same for the application. Additionally, users should be able to find for files based on the content, which can be helpful in refine the scope of the search and make it more accurate.
-
Highlight: Similar to a Google search engine, finding of files becomes faster!
-
Credits: Apache PDFBox® library ( PDDocument, PDFTextStripper )
-
-
move
command-
Description: I added the ability to move files within the application to any permissible directory within the user’s local device.
-
Justification: PDF++ is intended to be complete replacement for managing files. The user should be able to perform all the desired actions regarding file management through the application.
-
Highlight: When moving a file to a deeply nested directory, it is much more convenient as compared to manually navigating to the directory to move the file.
-
-
filter
command-
Description: I added the ability to filter files within the application based on the file tag(s) specified.
-
Justification: For files that are tagged, it is necessary to have a means to use the tags as identification - if not then tags would be purely aesthetic!
-
Highlight: Filtering files by tag allows you to group files strategically within the application, which would make file management much more convenient.
-
-
delete
command optimisation-
Description: Based on the original ability of addressbook to delete files recorded within the application (but not actually deleting he file from the local filesystem), I upgraded it so that there is an option for the user to delete the file both from the application as well as from the local filesystem.
-
Justification: PDF++ is intended to be complete replacement for managing files. From a user perspective, the intention behind deleting a file might be to remove the file completely. Otherwise, the user might encounter additional inconvenience e.g. deleting a file to create a new file of the same name, only to be unable to because the old file is still physically present.
-
Highlight: There is flexibility in how you want to delete a file from the application, as well as the convenience of using CLI which has the potential to be faster than clicking on the file directly.
-
-
sort
command optimisation-
Description: Based on the original ability of addressbook to sort the files based on ascending order of name, I upgraded it so that the user can specify if the sort should be done in ascending or descending order.
-
Justification: It is an implicit requirement that sorting features should have the flexibility for sorting to be done in either ascending or descending order.
-
Highlight: Sorting ability becomes more robust and gives the user the flexbility to sort as desired.
-
Code contributions:
Please click on any of these links to see samples of my code:
(v1.4) Merge command
(v1.3) Upgrade find command
(v1.3) Filter command
Documentation / Design contributions:
-
Developer Guide
-
Added detailed documentation for the following sections under Implementation to give a comprehensive view of the feature design, execution process, as well as any additional design considerations:
-
Merge Feature
-
Move Feature
-
Open Feature
-
Delete Feature
-
-
Created UML Activity Diagrams for the following sections under Implementation:
-
Merge Feature
-
Add Feature
-
Rename Feature
-
Encrypt Feature
-
Decrypt Feature
-
Clear Feature
-
-
Created UML Sequence Diagram for the Merge Feature section under Implementation.
-
-
User Guide
-
Added a step by step walkthrough to guide the user through merging of files. The walkthrough includes detailed instructions as well as visual aids of the application interface.
-
Minor contributions:
-
Set up RepoSense configuration for the team on our GitHub repository.
-
Integrated a third-party library Apache PDFBox® to the project which allowed for additional features to be performed on PDF files (e.g. reading of content, merging multiple files).
Contributions to Developer Guide
The following sections are excerpts from my additions to the PDF++ Developer Guide (continued next page):
UML Diagrams
Merge Command Activity & Sequence Diagrams
The aforementioned diagrams have been included in [Documentation] above respectively under Merge Feature.
Contributions to User Guide
The following is an excerpt from my additions to the PDF++ User Guide (continued next page):